Basically, if anyone is following this blog I wanted to let you know I am moving to Tumblr. I am changing the focus of my blog to be more about design, drawing, Ruby, Rails and things like that. Seemed like something to try and I have a friend in the business using Tumblr and find I agree with him a lot of things... so why not?
You can now find me at: http://www.aninvisiblefriend.com/
Thanks for reading!
Coding Kensho
Saturday, May 14, 2011
Friday, October 8, 2010
Slacker Duck Typing for Properties in Java
So the whole "walking, quacking, and giving advice on accident insurance" thing that ducks are know to do is not only a "cool" thing but a sanity thing. Once you have written your Nth DTO to Domain Object transformer, you get the feeling that you need some kind of framework to solve this problem. Why do you even need transformers? Well glad you asked. Also, what about that title, how does a transformer relate to Duck Typing? I'll get to that RSN.
Why Transformers?
Well it turns out that sometimes in Java land, where typing is static and strict, you need to deal with an ungainly domain object in sane, little pieces. Imagine a "roll-up" inheritance scenario where one DB row is N classes (with a type selector column). Imagine you have logic that wants to deal with specific types. What is an easy way to get that data from the blob to the type of interest?
Slacker Duck Typing
Given our problem statement, here is my suggestion for using BeanUtils to implement what I like to call Slacker Duck Typing. Let's assume we have a blobby type called Thingy, which for some reason is an instance of roll-up inheritance and is declared like this (I have omitted the getter/setters for each class to save space):
Now, imagine that you need to unpack the blob class and you want to unpack it into the correct real type. You'll notice I did not use inheritance because that would be wrong. LSP would be violated if I asked a human for their
What we need here is Duck Typing, we need to be able to assume if a class has some behavior (methods) then we should not care about the type and just invoke the methods. In our case, Human has a
Now, here's the slacker part shown in the
Did you see how lazy I was? I simply used:
to empower my program to extract property name-value pairs from one type and apply them to the other type. The best part for me is this, no one cares about types in this process because the transform logic, now deputized with the powers of BeanUtils, will happily get all the data in one object and apply it to another. If.... if you follow naming conventions (type conventions not required though I did use them in this example).
Now, this relies on BeanUtils not being too fussy about the types of data pushed to and pulled from the Map, but if it ever decided to do something that broke this, it is pretty easy to replace this code with your own.
So we see by being lazy and conventional (the names) we can do more work with less effort. At this point I must thank JBrains for making me aware of the power of BeanUtils and I'll thank some blobby code I had to deal with for inspiring me to think of using BeanUtils this way.
Why Transformers?
Well it turns out that sometimes in Java land, where typing is static and strict, you need to deal with an ungainly domain object in sane, little pieces. Imagine a "roll-up" inheritance scenario where one DB row is N classes (with a type selector column). Imagine you have logic that wants to deal with specific types. What is an easy way to get that data from the blob to the type of interest?
Slacker Duck Typing
Given our problem statement, here is my suggestion for using BeanUtils to implement what I like to call Slacker Duck Typing. Let's assume we have a blobby type called Thingy, which for some reason is an instance of roll-up inheritance and is declared like this (I have omitted the getter/setters for each class to save space):
public class Thingy {Further assume we have these two types: Human and Robot:
private String type;
private String name;
private String[] dna;
private Integer numOfCores;
public Thingy(String type, String name, Integer numOfCores, String[] dna) {
this.type = type;
this.name = name;
this.numOfCores = numOfCores;
this.dna = dna;
}
// getter/setter pairs were not put here to save space.
}
public static class Human {
private String name;
private String[] dna;
// getter/setter pairs were not put here to save space.
}
public static class Robot {
private String name;
private Integer numOfCores;
// getter/setter pairs were not put here to save space.
}
Now, imagine that you need to unpack the blob class and you want to unpack it into the correct real type. You'll notice I did not use inheritance because that would be wrong. LSP would be violated if I asked a human for their
numOfCores
or a robot for their dna
. Also, Java won't let me narrow the scope of the property methods of Thingy. What we need here is Duck Typing, we need to be able to assume if a class has some behavior (methods) then we should not care about the type and just invoke the methods. In our case, Human has a
name
and it has dna
which not-so-coincidently so does Thingy. Hmmm, so let's follow the rule that if the types cannot be related at least let the property names be the same.Now, here's the slacker part shown in the
main
:
public static void main (String[] args) throws InvocationTargetException, NoSuchMethodException, IllegalAccessException {
Thingy thingy = new Thingy("H", "Carbon Unit 100", null, new String[]{"CTGA", "CTTG", "AGTC"});
Map humanThingy = describe(thingy);
Map robotThingy = describe(new Thingy("R", "Joe", 10, null));
Human human = new Human();
populate(human, humanThingy);
String humanString = ToStringBuilder.reflectionToString(human).toString();
System.out.print(String.format("Humans are like this --> %s\n", humanString));
Robot robot = new Robot();
populate(robot, robotThingy);
String robotString = ToStringBuilder.reflectionToString(robot).toString();
System.out.print(String.format("Robots are like that --> %s\n", robotString));
}
Did you see how lazy I was? I simply used:
import static org.apache.commons.beanutils.BeanUtils.*;
to empower my program to extract property name-value pairs from one type and apply them to the other type. The best part for me is this, no one cares about types in this process because the transform logic, now deputized with the powers of BeanUtils, will happily get all the data in one object and apply it to another. If.... if you follow naming conventions (type conventions not required though I did use them in this example).
Now, this relies on BeanUtils not being too fussy about the types of data pushed to and pulled from the Map, but if it ever decided to do something that broke this, it is pretty easy to replace this code with your own.
So we see by being lazy and conventional (the names) we can do more work with less effort. At this point I must thank JBrains for making me aware of the power of BeanUtils and I'll thank some blobby code I had to deal with for inspiring me to think of using BeanUtils this way.
Saturday, October 2, 2010
Heroku the Great
Deployment Tears Be Gone...
It is true that using Heroku made my pains go away. My "I am doing this side project - this ultra lean startup and I don't have time for this" pains went away. It is simple. However, before walking in the door at Heroku you still need to assess your intentions.
How Are You Being Served?
This question is more about your use of Heroku as your customers see it. Ask yourself - how is this application being reached? Is it a stand alone? Is it one of those Facebook apps hosted here with data per user? Is it for a company? Is it SAS for many companies? Is it a hybrid?
My interest lies in a corporation using our application to add some capability to their web site for their own users. It is in effect SAS but serving the UI not just SOA services.
Serving many groupings of end users from one application means doing one of two things per Heroku Support (I asked after exhausting the docs and my patience searching at large):
It is true that using Heroku made my pains go away. My "I am doing this side project - this ultra lean startup and I don't have time for this" pains went away. It is simple. However, before walking in the door at Heroku you still need to assess your intentions.
How Are You Being Served?
This question is more about your use of Heroku as your customers see it. Ask yourself - how is this application being reached? Is it a stand alone? Is it one of those Facebook apps hosted here with data per user? Is it for a company? Is it SAS for many companies? Is it a hybrid?
My interest lies in a corporation using our application to add some capability to their web site for their own users. It is in effect SAS but serving the UI not just SOA services.
Serving many groupings of end users from one application means doing one of two things per Heroku Support (I asked after exhausting the docs and my patience searching at large):
- Create a separate deployment for each company - so you in effect are managing, paying for and billing for the cost of multiple applications on Heroku. There are no tools yet to automate that.
- Partition your data by corporation using it.
Tuesday, December 1, 2009
Art or Craft?
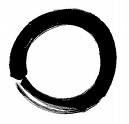
These days many of the programming legions are upon us with new criteria we have to meet to play "their" reindeer games. Let's say I don't care, let's say I care more about the art than the craft. For that is really true for me.
What's the difference? Well look at this picture, to the left. You know what it is right? It's a circle right? But it's not exactly a circle is it? It is also not totally finished and it is not wrong. It is unique and organic.
So, what does it matter? It is a circle for most of us and it is more. It is personal and is a stamp of identity of its creator. If you asked them to tell you how to create such a thing you might not understand the answer and you might even think they were being evasive.
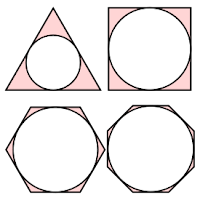
To do it requires you to master some tools for drawing, measurement and so forth. You need to adjust for changes in the drawing tools and medium. To me it is craft, the craft of drafting that comes to bear here.
I certainly accept one can have and benefit from a teacher in learning such a craft (in fact in high school I took a drafting class and benefitted from having a teacher). I admit there are masterful tricks and approaches. However, there is no way I will be convinced that one can realize the first image, the organic one, using the tools of the second example.
Okay then where am I going with this? Well, as I write this I see something like "islands of order" (craft) within the "ocean of chaos" (art). When we put together little bits like DTOs, DAOs, Facades and Adapters we are "craftsmen" (like plumbers in fact). When we go up higher and see the interfaces and user interfaces and the whole heaving ocean of parts that make up our application we are "artists".
Artists, especially ones who paint images like my first example are beyond myriad details. They see things beyond the well honed arcs and angles. Craftsmen are down at it, doing each part with polish, pattern and often a good bit of a priori knowledge of how all the parts will fit. They have a blueprint.
I have experienced two kinds of coding:
- Emergent - "Artistic" code which comes from somewhere in the back of my head.
- Convergent - "Crafted" code based on patterns I already saw and for which I could tell a detailed story.
The emergent kind came from my 10000 ft. flight in my mind over our problem space. The convergent came from detailed reduction.
We all have "flavors" or perspectives if you will, and around this center we inscribe our own "circles". I prefer the art to the craft. I prefer the free which is based on high level principles to the more precise and crafted. I like to experience the snarling tendrils of systems as they grow, wither and curl. When am I done? How about now?
It is personal and it is a preference, and even in the face of a movement trying to define the "right" way to be... I will be an artist (of sorts) who writes code. You can be what you want, just please don't be a pain in my a$$ about it.
Thursday, August 13, 2009
No Function Overloading? Not a Problem.
Recently, as I got back to Ruby, or should I say got back learning more about Ruby after skirting the language (in depth) and still getting Rails coding done... I decided I needed to kick it up a notch and get Rubier. I enjoy this task, because I can focus and think.
My source for this focus is The Well Grounded Rubyist and while the book has a lot of OO-newbish stuff I find the succinct portions readable and so I skim and enjoy the book. Anyway, one of the things that came up while reading it was the explanation of something that trips up most former C++ and Java programmers jumping into Ruby - no overloading (at least not the way that Java and C++ do it)!
Seems like heresy, for an OO language right? However, Ruby can realize the same concepts:
However, I want to point out something I have done even when programming in Java or C++: I have not not used overloading. I started realizing the value of this approach working with some Math whiz who was helping me discuss an API design. For him, overloading was a clever parlor trick that did nothing to tell the person reading your code what you mean by supporting n-ways to call the same logic - without extra documentation.
His suggestion? Name the overloaded functions to tell a story:
If you name methods explicitly and you encounter a function invocation like this:
You have a real good idea what the function is doing and also why it needs the data that you give it. In the overloading case its not just black box implementation - which is a good thing we call encapsulation; it is also black box semantics, which is not good in any case and requires yet more data to untangle the intentions.
Encapsulation should protect implementations, not obscure semantics. In programs, clear intentions are what reduce the occurrence of the Lava Flow Anti-pattern or inadvertent deletion by refactoring to "clean up the junk".
I have used overloading but in the end I think it is less OO than some assert, I also think it is a bit of a cheap trick. Most importantly, I know it can obscure semantics.
So for me, Ruby "not having overloading" (like Java or C++) is not a problem. In fact, what I just said about clarity of semantics also applies to Ruby's "overloading" by default arguments pattern. Maybe no public function should leverage that trick either. Perhaps it's just a tool to help keep the internals clean and lean?
My source for this focus is The Well Grounded Rubyist and while the book has a lot of OO-newbish stuff I find the succinct portions readable and so I skim and enjoy the book. Anyway, one of the things that came up while reading it was the explanation of something that trips up most former C++ and Java programmers jumping into Ruby - no overloading (at least not the way that Java and C++ do it)!
Seems like heresy, for an OO language right? However, Ruby can realize the same concepts:
- Ruby has default values for arguments so if you construct your parameter list correctly, you can realize overloading.
- If you need optional arguments as part of the overloading option - Ruby has the optional argument support.
However, I want to point out something I have done even when programming in Java or C++: I have not not used overloading. I started realizing the value of this approach working with some Math whiz who was helping me discuss an API design. For him, overloading was a clever parlor trick that did nothing to tell the person reading your code what you mean by supporting n-ways to call the same logic - without extra documentation.
His suggestion? Name the overloaded functions to tell a story:
- Overloaded: calc_net_pay(tax_rate, the_401k_rate) and calc_net_pay(tax_rate)
- Informative: calc_net_pay_for_specific_401K_rate(tax_rate, the_401k_rate) and calc_net_pay_for_default_401K_rate(tax_rate)
If you name methods explicitly and you encounter a function invocation like this:
calc_net_pay_for_specific_401K_percent(get_employee_tax_rate(), get_employee_401_rate())
You have a real good idea what the function is doing and also why it needs the data that you give it. In the overloading case its not just black box implementation - which is a good thing we call encapsulation; it is also black box semantics, which is not good in any case and requires yet more data to untangle the intentions.
Encapsulation should protect implementations, not obscure semantics. In programs, clear intentions are what reduce the occurrence of the Lava Flow Anti-pattern or inadvertent deletion by refactoring to "clean up the junk".
I have used overloading but in the end I think it is less OO than some assert, I also think it is a bit of a cheap trick. Most importantly, I know it can obscure semantics.
So for me, Ruby "not having overloading" (like Java or C++) is not a problem. In fact, what I just said about clarity of semantics also applies to Ruby's "overloading" by default arguments pattern. Maybe no public function should leverage that trick either. Perhaps it's just a tool to help keep the internals clean and lean?
Friday, July 3, 2009
Pen v. Sword and now Pen v. Web
A Word From Our Sponsor
Today's post is more about an emerging pattern and a success in applying it vs. a struggle in applying it that is ongoing. I will start out by saying I am not naming the other parties in the "struggle" because those negotiations are under way.
Success
What I am about to share with you is a link to a web site which The Art of Agile Development which is a very useful book, but it is still paper. It cannot help you model or guide you. Sure I love books, I have more than I can read, and surveys, profiles, and tests are useful. They are more useful online.
My friend Sebastian Hermida implemented what I feel is a great tool and the author feels is an awesome viral marketing tool for The Art of Agile Development. In fact, providing such a tool like his Better Team Site free, leverages the principle reciprocity that can benefit sales of the book.
The Struggle
The struggle I face is convincing a publisher that the same thing will help their book - viral marketing and reciprocity. The author has been pleading on my behalf and now it is down to me to leverage my influence skills to get some kind of web site using a survey from the book - a useful survey I might add.
So wish me luck, I am obviously one of the first people this publisher has encountered asking for such permission. What year is it again? 2009? Really?
Today's post is more about an emerging pattern and a success in applying it vs. a struggle in applying it that is ongoing. I will start out by saying I am not naming the other parties in the "struggle" because those negotiations are under way.
Success
What I am about to share with you is a link to a web site which The Art of Agile Development which is a very useful book, but it is still paper. It cannot help you model or guide you. Sure I love books, I have more than I can read, and surveys, profiles, and tests are useful. They are more useful online.
My friend Sebastian Hermida implemented what I feel is a great tool and the author feels is an awesome viral marketing tool for The Art of Agile Development. In fact, providing such a tool like his Better Team Site free, leverages the principle reciprocity that can benefit sales of the book.
The Struggle
The struggle I face is convincing a publisher that the same thing will help their book - viral marketing and reciprocity. The author has been pleading on my behalf and now it is down to me to leverage my influence skills to get some kind of web site using a survey from the book - a useful survey I might add.
So wish me luck, I am obviously one of the first people this publisher has encountered asking for such permission. What year is it again? 2009? Really?
Wednesday, June 17, 2009
Rails pre-2.3 and Single Form Object Graph Pains
The Problem
Recently, I was working on part of a Rails application for which I found myself needing to use polymorphic relationships and I wanted to give value objects a try.
Since the code is something under development, I will make up a simple case here. Keep in mind some goals:
My Approach - Do Next to Nothing
The reason for this approach is to do nothing extra to get this function done and do the right thing when I take on Rails 2.3. Of course, I may decide the Presenter is the way to go.
The above resources gave me an no immediate answer for the Value Objects - these turned out to give me various problems which I began to realize required more view supporting fake model attributes to handle param hashes for the Value Objects. Another option presented by this blog involved some stubbed out methods to make use of ActiveRecord:Validations and that made me feel like I was getting a little off track.
So I ended up with:
Contact Info has a denormalized set of attributes - actually that is not totally true but lots of people do tend to break Phone and Address down into tiny pieces and distinct tables. I did not do so and at this point you will notice my value object for phone has one attribute in use at this time - I am planning to using the other attributes later.
As of now, I am only supporting create processing and I will show parts of the partial I use to render this data:
Here we show both an attribute from the parent (Investor) object and the child (ContactInfo) object. We get around the whole pain with forms from using Value Objects by mapping to the attributes for the Investor and ContactInfo objects and not the Value Objects. No I don't love it, but I prefer it to the alternatives. Less code for me to write and looking to Rails 2.3 to fix this.
Okay, so you see @investor and @contact_info right? I maintain them as member variables of the investor controller. Here is the create method I use and save transaction. I took this approach to avoid saving the parent if there were errors in the child because the form treated the data as one entity - like an updateable view in a database.
I admit I still need to go back and tweak the format.xml section for @contact_info errors, my immediate need for forms to work is met. I am not overjoyed at this code, it works and I used Rails out of the box to do it.
I still think maybe Presenter is a better bet, but that is a refactoring adventure for another time.
Recently, I was working on part of a Rails application for which I found myself needing to use polymorphic relationships and I wanted to give value objects a try.
Since the code is something under development, I will make up a simple case here. Keep in mind some goals:
- The polymorphic child is meant to reduce a common concept to one table - so I want to have Rails help me track the type of parent using it.
- I used value objects to decompose the polymorphic child into usable chunks - at this point I had little interest in breaking the subparts into tiny, annoying tables. Though by leveraging value objects now, I get to defer that decision all while using a healthy decomposition.
- One form - with several subsections.
My Approach - Do Next to Nothing
The reason for this approach is to do nothing extra to get this function done and do the right thing when I take on Rails 2.3. Of course, I may decide the Presenter is the way to go.
The above resources gave me an no immediate answer for the Value Objects - these turned out to give me various problems which I began to realize required more view supporting fake model attributes to handle param hashes for the Value Objects. Another option presented by this blog involved some stubbed out methods to make use of ActiveRecord:Validations and that made me feel like I was getting a little off track.
So I ended up with:
- A controller managing multiple object reference variables.
- Using a transaction block.
- Not referring to my Value Objects in my form - but I kept them in the object because I hope to use them later.
class Investor < on =""> :save, :message => "can't be blank"
validates_associated :contact_info, :on => :save
composed_of :name, :mapping => [%w(contact_full_name, full_name)]
has_one :contact_info, :as => :contactable
end
Contact Info has a denormalized set of attributes - actually that is not totally true but lots of people do tend to break Phone and Address down into tiny pieces and distinct tables. I did not do so and at this point you will notice my value object for phone has one attribute in use at this time - I am planning to using the other attributes later.
class ContactInfo < on =""> :save, :message => "can't be blank"
validates_presence_of :mailing_city, :on => :save, :message => "can't be blank"
validates_presence_of :mailing_state, :on => :save, :message => "can't be blank"
validates_presence_of :primary_number, :on => :save, :message => "can't be blank"
validates_presence_of :contactable, :on => :save, :message => "can't be unvalued"
composed_of :mailing_address, :class_name => "Address", :mapping => [ %w(mailing_address1 address1), %w(mailing_city city), %w(mailing_state state), %w(mailing_zip_code zip_code), %w(mailing_address2 address2) ] do |params|
Address.new params[:mailing_address1], params[:mailing_city], params[:mailing_state], params[:mailing_zip_code], params[:mailing_address2]
end
composed_of :primary_phone, :class_name => "Phone", :mapping => [ %w(primary_number number) ] do |params|
Phone.new params[:primary_number]
end
belongs_to :contactable, :polymorphic => true
end
class Address
attr_reader :address1, :address2, :city, :state, :zip_code
def initialize(address1, city, state, zip_code, address2 = nil)
@address1, @address2, @city, @state, @zip_code = address1, address2, city, state, zip_code
end
end
class Phone
attr_reader :number, :extension
def initialize(number, extension = nil)
@number, @extension = number, extension
end
end
class Name
attr_reader :full_name
def initialize(full_name)
@full_name = full_name
end
end
As of now, I am only supporting create processing and I will show parts of the partial I use to render this data:
<% form_for @investor do |f| -%>
<div class="data_sub_section">
<p>
<%= f.label :contact_full_name, "Full Name: ", :class => "edit_required_label" %>
<%= f.text_field :contact_full_name, :class => "data_field" %>
</p>
<..... excerpt ......>
<% fields_for @contact_info do |f_contact_info| -%>
<div class="data_sub_section">
<p>
<%= f_contact_info.label :mailing_address1, "Address1: ", :class => "edit_required_label" %>
<%= f_contact_info.text_field :mailing_address1, :class => "data_field" %>
</p>
<..... excerpt ......>
Here we show both an attribute from the parent (Investor) object and the child (ContactInfo) object. We get around the whole pain with forms from using Value Objects by mapping to the attributes for the Investor and ContactInfo objects and not the Value Objects. No I don't love it, but I prefer it to the alternatives. Less code for me to write and looking to Rails 2.3 to fix this.
Okay, so you see @investor and @contact_info right? I maintain them as member variables of the investor controller. Here is the create method I use and save transaction. I took this approach to avoid saving the parent if there were errors in the child because the form treated the data as one entity - like an updateable view in a database.
def create
@investor = Investor.new(params[:investor])
begin
Investor.transaction do
@contact_info = ContactInfo.new(params[:contact_info])
@contact_info.contactable = @investor
@investor.contact_info = @contact_info
@investor.save!
end
all_valid = true
rescue ActiveRecord::RecordNotSaved, ActiveRecord::RecordInvalid
all_valid = false
end
respond_to do |format|
if all_valid
flash[:notice] = 'Investor was successfully created.'
format.js
format.xml { render :xml => @investor, :status => :created, :location => @investor }
else
flash[:notice] = 'Investor was not created.'
format.js
format.xml { render :xml => @investor.errors, :status => :unprocessable_entity }
end
end
end
end
I admit I still need to go back and tweak the format.xml section for @contact_info errors, my immediate need for forms to work is met. I am not overjoyed at this code, it works and I used Rails out of the box to do it.
I still think maybe Presenter is a better bet, but that is a refactoring adventure for another time.
Subscribe to:
Posts (Atom)